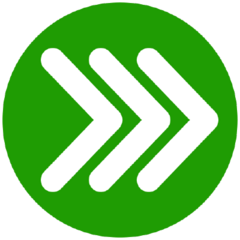
psaverse
psaverse.Rmd
Setting up a PSA Project Package
First, you would need to run the psa_create_project()
function including the path you want to start the folder on your
computer and the name of the project. After you run this function, the
new package R project will open automatically for you.
psa_create_project(
path = "~/Github", # path you want this on your computer
psa_name = "PSA007-spaml" # name of the project
)
Create/Update Descriptions
You can update the description of the package using the
psa_update_description()
function.
psa_update_description(
package_title = "SPAML IS GREAT",
description = NULL,
version = NULL,
license = NULL
)
You can add authors to the package for other project monitors or the lead author of the paper.
Create Folder Structure
psa_create_folder("all")
will create the entire folder
structure for the package, while you can also add one at a time.
Other options:
- “ethics”: creates the “01_Ethics” folder.
- “power”: creates the “02_Power” folder.
- “materials”: creates the “03_Materials” folder.
- “procedure”: creates the “04_Procedure” folder.
- “data”: creates the “05_Data” folder.
- “analysis”: creates the “06_Analysis” folder.
- “communication”: creates the “07_Communication folder.
- “all”: creates all eight main folders.
- Any other parameter option will be added into the 08_Other folder.
# will create all folders
psa_create_folder("all")
psa_create_folder("cheese")
# make sure this works in markdown as well as regular script
Add Documents
You can then manually move the files you need into the folder
structure or use the psa_add_documents()
function to add
individual files or entire folders.
psa_add_documents(
folder = "ethics", # where should it go
path = "~/Downloads", # to a single file or directory
should_replace = FALSE, # overwrite existing files?
recursive = FALSE # better explain this in docs
)
# recheck copying individual files
# path should allow vector of file paths like a list of PDFs
# or a directory
# or single file
Add R Package Dependencies
After you add your R scripts in .R
or .Rmd
format, you can use a convenience function to add all libraries that can
be found in those scripts. The default is to examine all files in the
inst
folder and add those packages.
psa_dependencies(
scan_folder = "inst",
add_package = NULL # has to be one at a time
)
# add qmd files
# allow for vector of packages to be added
Add this Package to PSA GitHub
First, you should confirm you have the ability to push to the PsySciAcc github organization at https://github.com/psysciacc. Please ask to be added to the team.
Second, you can use the psa_git()
function to initialize
your new project as a github repository associated with the PsySciAcc
organization. You can then use your favorite way to github to push and
pull to update the package. The default is to create a private
repository until you are ready to release the project.
psa_git()
Check Package Folders
This function will check that you meet the minimum requires for documentation and files for the PSA folder structure. You will receive information on what you are missing.
Options: “ethics”, “power”, “materials”, “procedure”, “data”, “analysis”, “communication”, “all”.
psa_check_folder(folder = "ethics")
# be sure to set working directory to the project so make it a global function
working folder should not be empty if so make it “.”
can’t write the testthat (or subfolders) for CRAN, so make sure it’s writing to a temp directory (check scienceverse)
https://scienceverse.github.io/scienceverse/index.html https://github.com/scienceverse/scienceverse/
https://github.com/debruine/booktem/blob/master/tests/testthat/test-create_book.R - preclean up - and then if stops be sure to clean up as well